How to generate a website screenshot with Snapsbrew's API
To be able to successfully complete this guide you'll need to have an active Snapsbrew account, if you don't have one you can sign up for free right here
Introduction
A picture is worth a thousand words—or in this case, clicks. Screenshots are an easy and effective way to show people what your page or product looks like without them having to click through.
The nice thing is that you can control the message. By framing the screenshot in a certain way, you can guide users' eyes to focus on the most important elements on the page and highlight key features that might not be immediately apparent otherwise.
Generating them with Snapsbrew's API is easy as pie.
How to connect to Snapsbrew's API
To connect to Snapsbrew you'll need to log into Snapsbrew's dashboard, and then go to your account page.
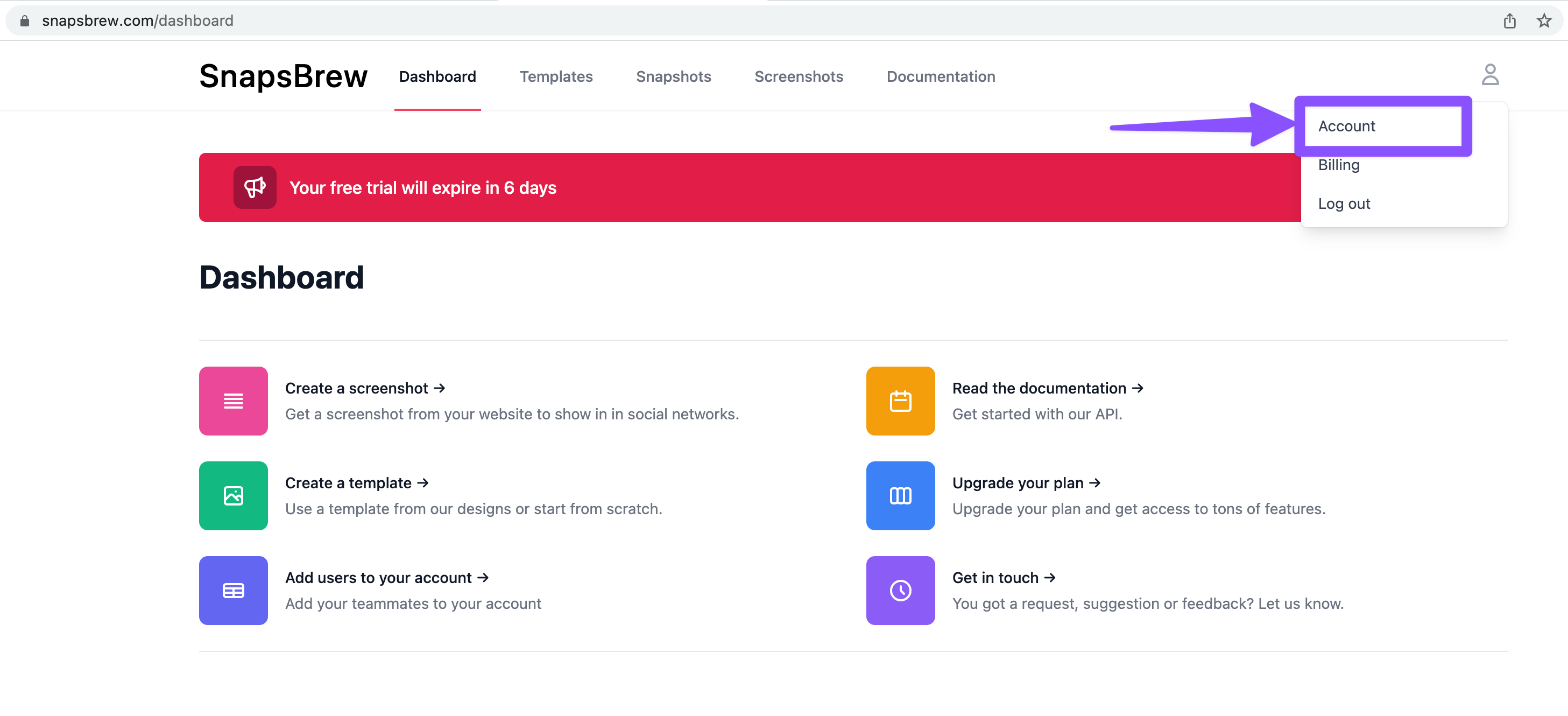
Right here you can generate a new API Token. You can generate as many as you want. Please keep in mind that these are only shown once and there's no way to recover them, so please make sure you store them in a secure place after generating them.
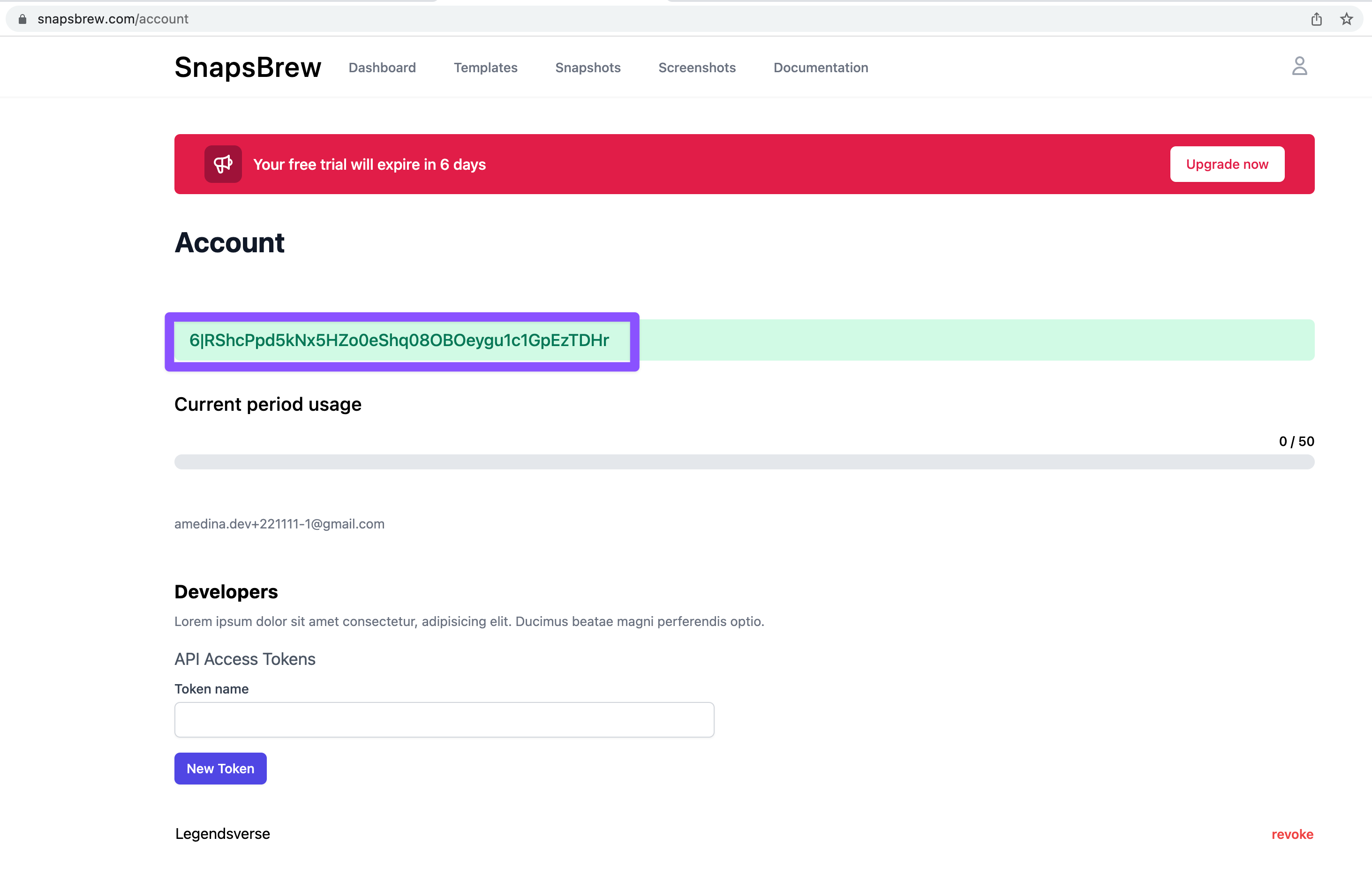
We'll use this token to make the API call, for this guide purposes we'll make the API call using Javascript's fetch API.
Setting up a screenshot API call
Let's start by declaring a few constants:
const url = 'https://snapsbrew.com/api/v1/snaps'
const apiToken = '4|s9fm3UleEXeE9gm3oVYYxRnfAtL0xzrvOKvVJ8PU'
const screenshotUrl = 'https://legendsverse.com'
Before setting up the fetch method in our script, let's talk about the payload we have to send to Snapsbrew's API. To take a screenshot all it takes is an `url` param, as simple as this:
const body = JSON.stringify({
"url": "https://legendsverse.com"
})
The nice thing about this endpoint is that you can also set any `width` and `height`, for example, if you want to take a screenshot of the size of an iPhone 12 Pro:
const body = JSON.stringify({
"url": "https://legendsverse.com",
"width": 390,
"height": 844
})
Now that we know the body payload we can set up the fetch method in our script.
const res = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiToken}`
},
body: body
})
How to make an API call to Snapsbrew
Now, all we have to do is call this function and wait for the magic to happen.
const url = 'https://snapsbrew.com/api/v1/snaps'
const apiToken = '4|s9fm3UleEXeE9gm3oVYYxRnfAtL0xzrvOKvVJ8PU'
const screenshotUrl = 'https://legendsverse.com'
async function generateScreenshot(url) {
const body = JSON.stringify({
"url": url
})
const res = await fetch(url, {
method: 'POST',
headers: {
'Content-Type': 'application/json',
'Authorization': `Bearer ${apiToken}`
},
body: body
})
const responseData = await res.json()
return responseData
}
const snapsData = await generateScreenshot(
'https://legendsverse.com'
)
And Ta-da! you have a screenshot of your website. 😎
Final thoughts
That's it for this guide, as you can see generating screenshots is easy as pie. I hope you found it useful and if you have any issues, concerns or things just don't work, feel free to reach me at [email protected] , and I will do everything in my power to help you
Take your marketing a step forward Start your free trial today.
Automate repetitive tasks that could be done by a computer. Focus on things only you can do.
Sign up for free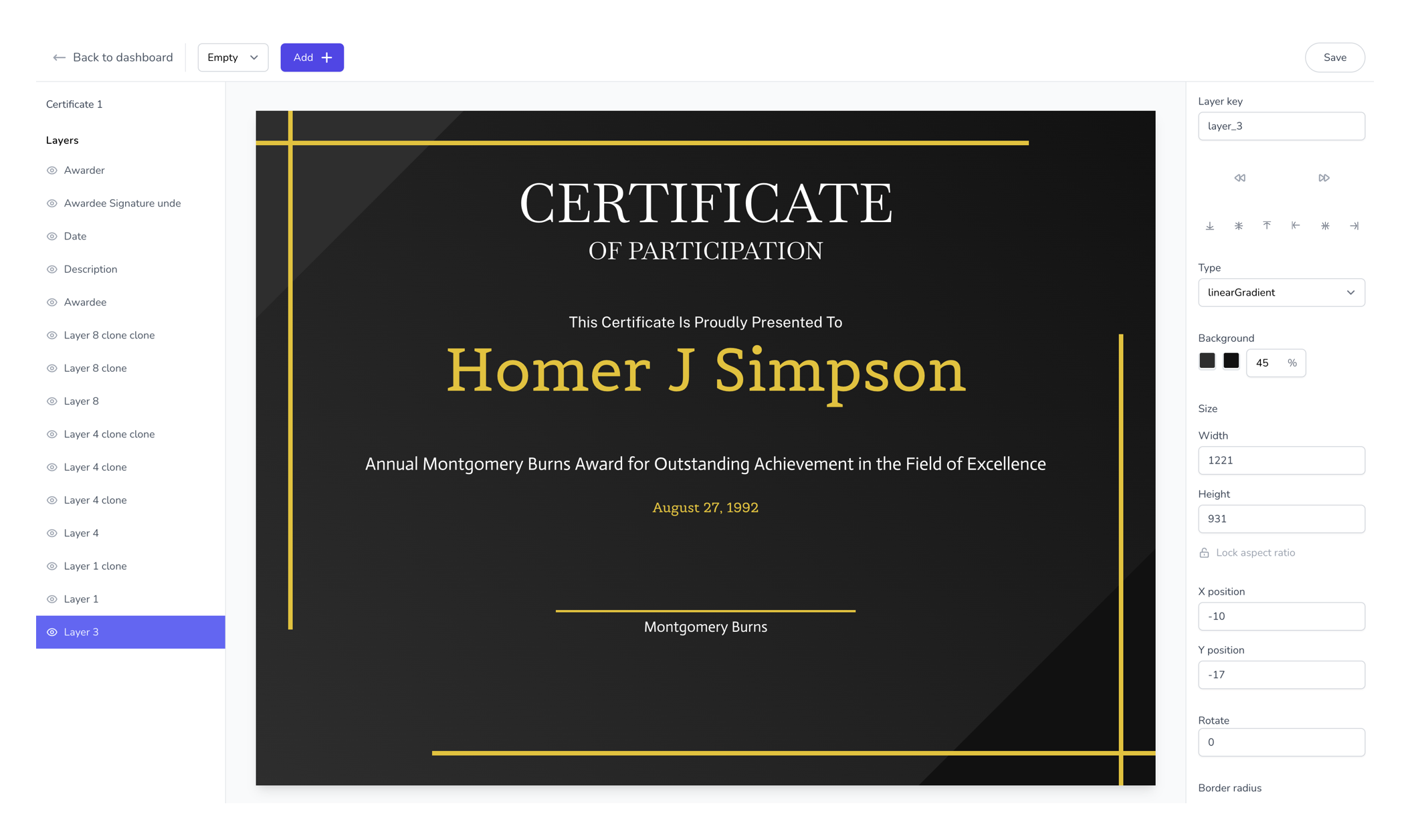